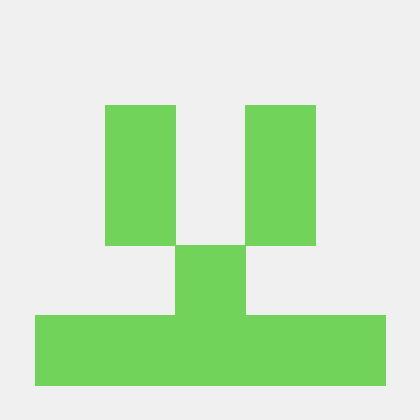
Dart Flutter Best Practices .cursorrules prompt file
About .cursorrules prompt file
What you can build
- Clean Dart Code Generator: A tool that generates Dart code snippets following the provided guidelines, ensuring clean programming with proper types, naming conventions, and Flutter principles.
- Dart Refactoring Assistant: An app that analyzes your Dart code and suggests refactorings to align with the clean programming principles, such as reducing nested widgets, separating logic into controllers, and encapsulating data.
- Visual Dart Function Tester: A website to create and run tests visually that adhere to the Arrange-Act-Assert and Given-When-Then conventions in Dart, enabling users to import their function code and design tests interactively.
- Flutter Architecture Advisor: A service that evaluates Flutter projects and provides insights or improvements based on clean architecture principles, suggesting module separations, state management using Riverpod, and optimal dependency injection with getIt.
- SOLID Class Designer: A design tool that assists in creating Dart classes aligned with SOLID principles. It provides guidance on class responsibilities, interface declarations, and focusing on single-purpose, small classes.
- Nested Widget Optimizer: An app specifically for refactoring deeply nested Flutter widgets by breaking them into reusable, focused components, enhancing both app performance and code readability.
- Constant and Theme Management System: A plugin that helps in managing constants and themes efficiently using the best practices outlined, like leveraging ThemeData or AppLocalizations for easily managing UI constants and translations.
- Dart Type Safety Enforcer: A tool that reviews Dart code and ensures type declarations for every variable, function, and object, discouraging the use of the 'any' type and promoting the creation of necessary types.
- Exception Handler Configurator: A service to set up and customize exception handling in Dart, suggesting best practices for catching exceptions, adding context, and global error handling.
- State Management Checker: A tool specifically for verifying and optimizing state management within Flutter apps using Riverpod, ensuring states are kept alive appropriately and managed effectively across the app's lifespan.
Benefits
- Emphasizes clean architecture and design patterns specific to Flutter, including the use of Riverpod for state management and repository pattern for data persistence.
- Advocates for strict Dart nomenclature, including PascalCase for classes and camelCase for functions/variables, ensuring code readability and consistency.
- Encourages breaking down complex structures into reusable components and smaller units, enhancing both performance and maintainability in Flutter app development.
Synopsis
Flutter developers could build efficient, clean, and maintainable mobile apps using the provided guidelines to ensure structured and scalable code.
Overview of .cursorrules prompt
The .cursorrules file provides a set of guidelines and conventions for Dart programming, particularly when using the Flutter framework. It emphasizes best practices in coding style, naming conventions, function design, data handling, class structure, and error handling. Specific guidelines are included for maintaining clean architecture in Flutter, managing state with Riverpod, and utilizing other frameworks and patterns such as the repository pattern, AutoRoute, and getIt. The file also covers testing strategies, including unit tests, acceptance tests, and widget testing for ensuring code quality and reliability. Overall, it aims to promote clean, efficient, and scalable code.