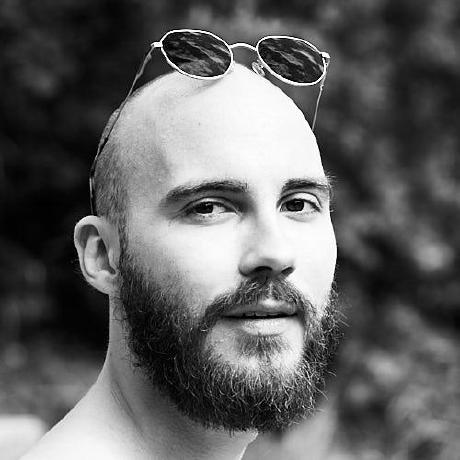
Rust Tokio Async Programming .cursorrules prompt file
About .cursorrules prompt file
What you can build
Concurrent Web Scraper: Develop a highly efficient concurrent web scraper using Rust's async capabilities, leveraging
tokio
for managing network requests and handling data concurrently. This tool can scrape multiple websites simultaneously with robust error handling and backpressure mechanisms.Async Task Scheduler: Create an async task scheduling application in Rust, allowing users to schedule, manage, and execute tasks asynchronously. Utilize
tokio::select!
for task cancellations andtokio::sync::oneshot
for communication between scheduled tasks and the central management system.Real-time Chat Application: Build a real-time chat application with Rust using websockets, incorporating
tokio
for async message handling. Implement channels (tokio::sync::mpsc
andtokio::sync::broadcast
) for efficient message distribution to multiple users.Async File Processing System: Develop a system for processing large volumes of files asynchronously. Use
tokio
for non-blocking I/O operations and implement queues withtokio::sync::mpsc
to parallelize file processing tasks.High-performance HTTP Server: Design a high-performance HTTP server using Rust and
hyper
, capable of handling numerous concurrent requests efficiently. Leveragetokio::spawn
to manage connections and ensure minimal latency with robust backpressure controls.Rust-based Microservice Framework: Create a framework for building microservices in Rust with complete async support, providing libraries for common operations like HTTP routing, database interaction with
sqlx
, and service discovery.Async Data Pipeline: Construct an async data processing pipeline that ingests data, processes it in parallel, and outputs it to storage like an async database using
tokio-postgres
. Implement bounded channels to handle flow control and back pressure.Smart IoT Device Manager: Develop a Rust-based IoT device management system, coordinating multiple devices asynchronously using
tokio
. Implement error handling strategies to maintain device connections and ensure reliable operations.Distributed Key-Value Store: Design a distributed key-value store using Rust's concurrency model, with async networking for replication and
tokio::sync::RwLock
for handling concurrent read-write operations.Async API Gateway: Build an async API gateway in Rust for handling large scale API requests, implementing load balancing, and asynchronous request forwarding with
serde
for payload management andreqwest
for external API calls.
Benefits
- Emphasizes modular code with Rust's idiomatic naming and strict adherence to ownership and concurrency principles.
- Prioritizes async management using
tokio
, ensuring robust task handling, structured concurrency, and effective resource management. - Comprehensive focus on testing, safety, and performance optimization tailored for async programming using industry-standard Rust libraries.
Synopsis
Rust developers building high-performance, scalable, and concurrent systems would benefit from this prompt to optimize their async Rust applications using `tokio`.
Overview of .cursorrules prompt
The .cursorrules file provides guidelines for writing Rust code with a focus on asynchronous programming and concurrency. It emphasizes using the `tokio` library for async task management and provides principles for maintaining clean, modular, and efficient code. Key topics covered include effective use of async functions, channels, error handling, and testing. It also highlights performance optimization techniques and best practices for structuring applications, as well as recommended libraries for networking, serialization, and database interactions within the async ecosystem.